Welcome to the tutorial on using the OpenAI package in Python for image manipulation! OpenAI is a cutting-edge artificial intelligence (AI) research organization that has developed a wide range of powerful tools and libraries, including one specifically designed for image manipulation. In this tutorial, we will explore how you can leverage the OpenAI package to perform various image manipulation tasks, such as generating images, modifying images, and enhancing images, all using the power of AI. Whether you are a seasoned Python developer or just getting started with image manipulation, this tutorial will provide you with step-by-step instructions and examples to help you harness the capabilities of the OpenAI package and create stunning visual effects in your images. We will be going over the three ways you can use their package to edit photos, that is, creating new images, editing images, or creating image variations. So let's dive in and unlock the potential of OpenAI for image manipulation!
Step 1-) Create API Key
You need an API key to access their API. Initially, the API key is free. Keep this key secure.
https://platform.openai.com/account/api-keys
Step 2-) Pip Install OpenAI
In a shell, run the command:
> pip install openai
Step 3-) Image Generation
The first part of their package simply allows you to generate images from descriptions, which is quite remarkable and surprisingly pretty accurate. The code we use is as follows:
import openai import constants # Set up the OpenAI API client openai.api_key = constants.API_KEY description = "a stained glass window with an image of fruits" # Generate a image from ChatGPT response = openai.Image.create(prompt=description, n=1, size="512x512") image_url = response["data"][0]["url"] # Print the response print(image_url)
The code does the following:
- Imports the package and import constants (which contain our API Key). You can paste the secret in text in the code but that is bad practice.
- We set the API key in the package and set the description for the AI
- We call the API by passings in the params, where n is the number of images and size is the size of the image. As of writing the API only accepts a set of square images.
- We get the URL from the API and open it.
The result I got looks as follows:
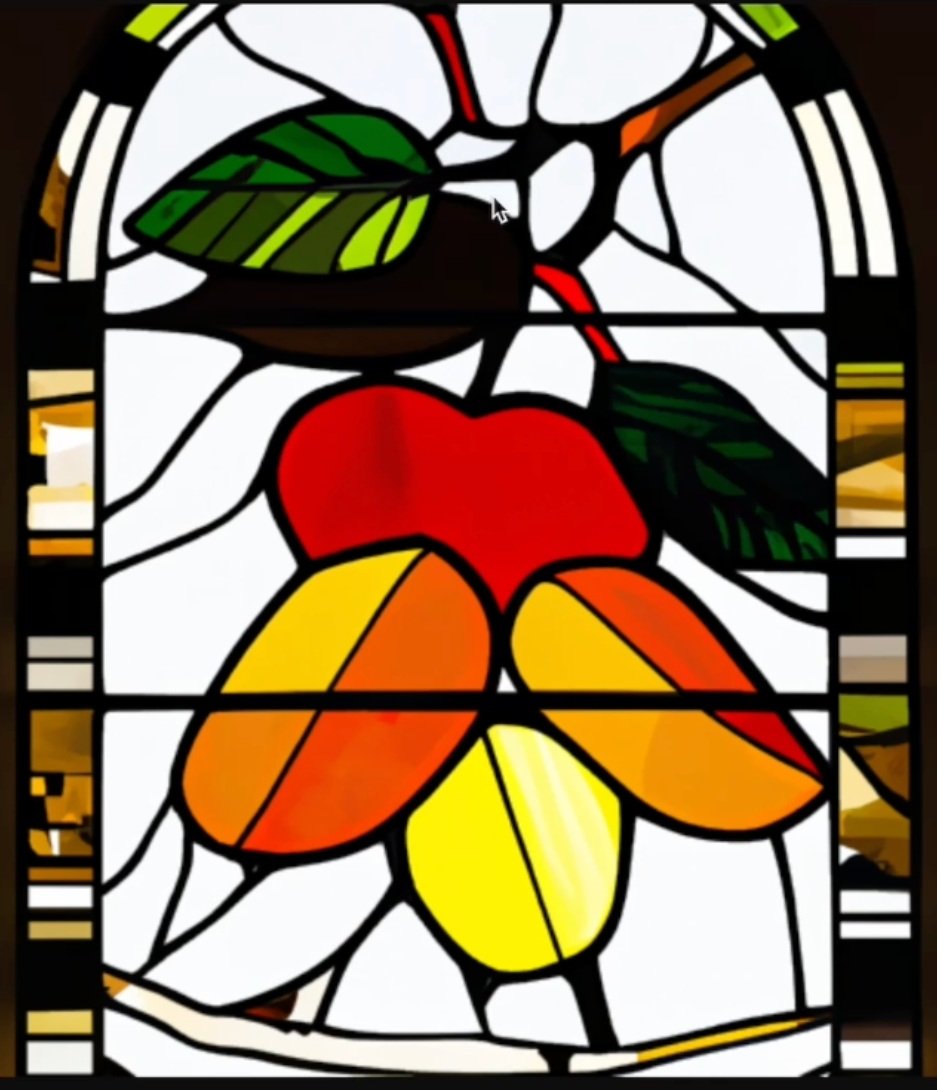
Pretty remarkable how it accurately matches the text description!
Note that the URL lasts a given amount of time (1 hour), so save it locally if you want to keep the image somewhere to show your friends or family.
Step 4-) Image Editing
You can also edit images with a mask using their package. This is my favorite part of the package because you can essentially photoshop a photo with an image, mask, and description. Here is the example I went over in the video.
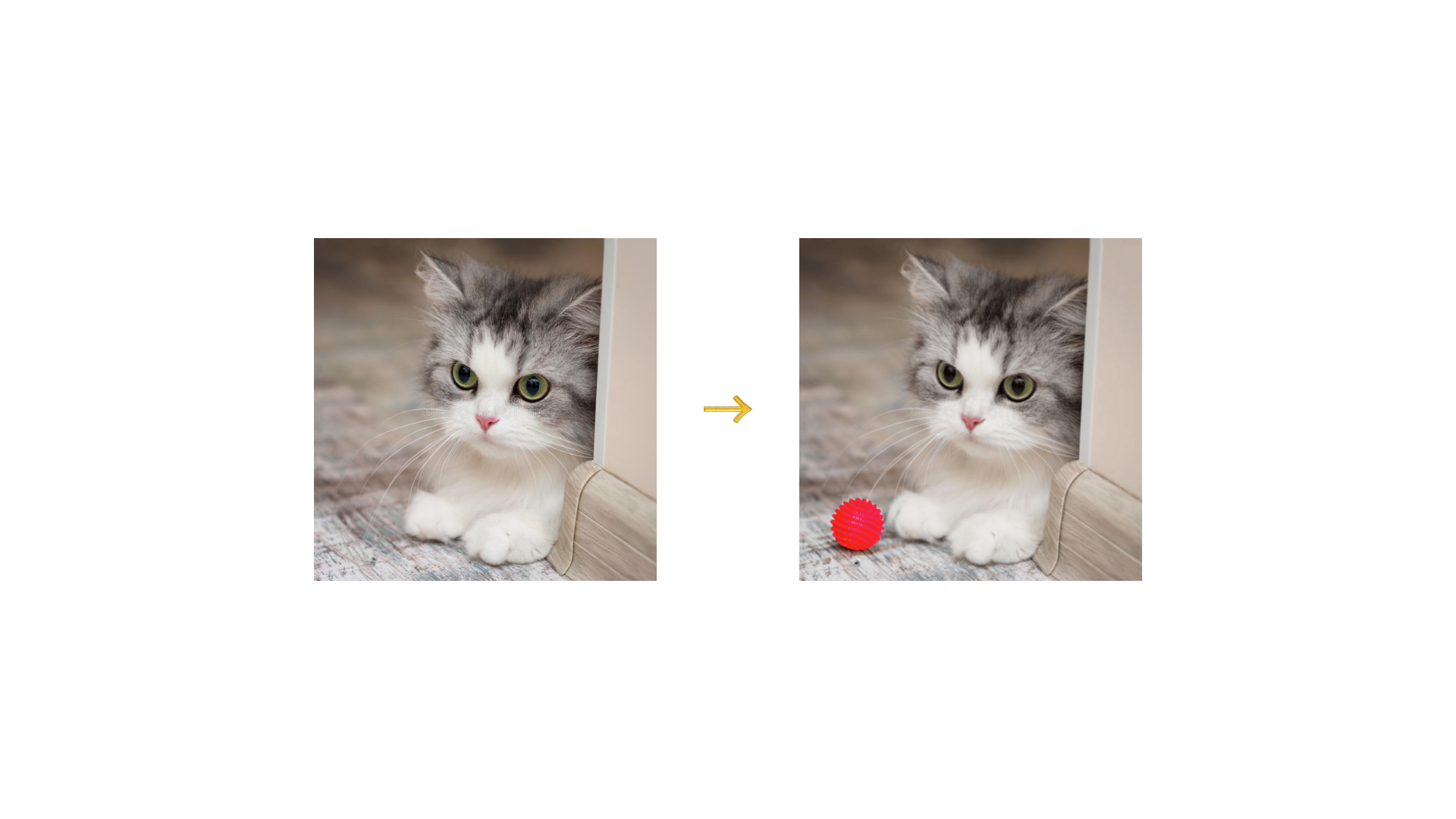
Here is the mask I used:
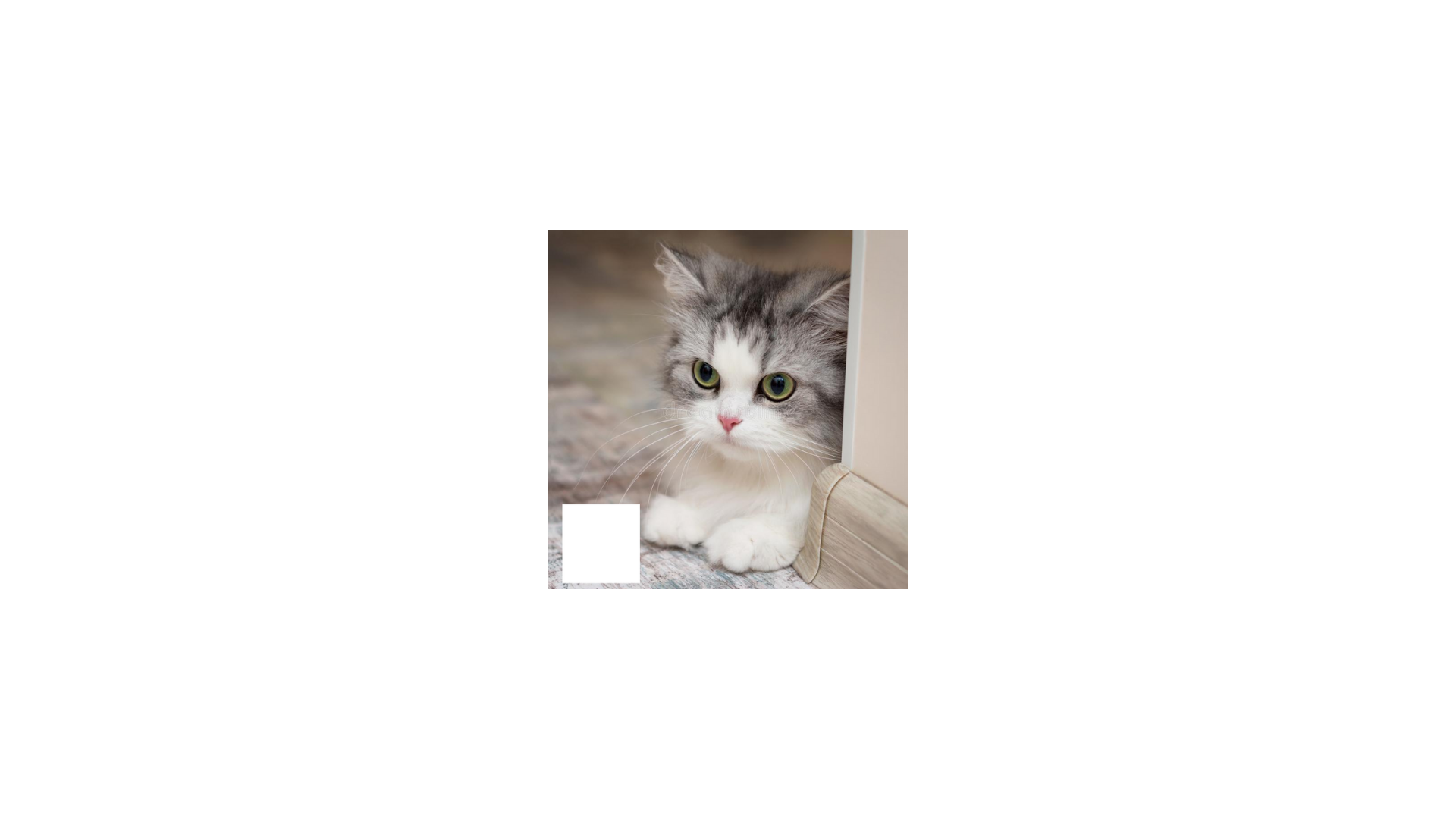
You can see that the AI added the ball in the transparent area of the mask.
Some important things to note:
- You need a square image and a square mask.
- You can create a mask of an image here using this free online tool. It only allows a square mask.
- You need to describe the image itself and what you want in the mask, the AI needs to have some context on the existing image, or else the edit will not work.
- The image I found online was in “Palette” mode, so I needed to initially convert it to RGB by using the Pillow library in Python. You can uncomment the code below if you are having Palette issues.
import openai # from PIL import Image import constants # Set up the OpenAI API client openai.api_key = constants.API_KEY # Image.open("dalle-2 examples/cute_cat.png").convert("RGB").save( # "dalle-2 examples/cute_cat.png" # ) # Generate a image from ChatGPT response = openai.Image.create_edit( image=open("dalle-2 examples/cute_cat.png", "rb"), mask=open("dalle-2 examples/mask.png", "rb"), prompt="A black and white cat with a red ball", n=1, size="512x512", ) image_url = response["data"][0]["url"] # Print the response print(image_url)
Step 5-) Image Variation
Finally, the simplest of the three is the image variation API they over. It simply allows you to pass an image and the AI creates a random variation in the image you provide. Here is an example.
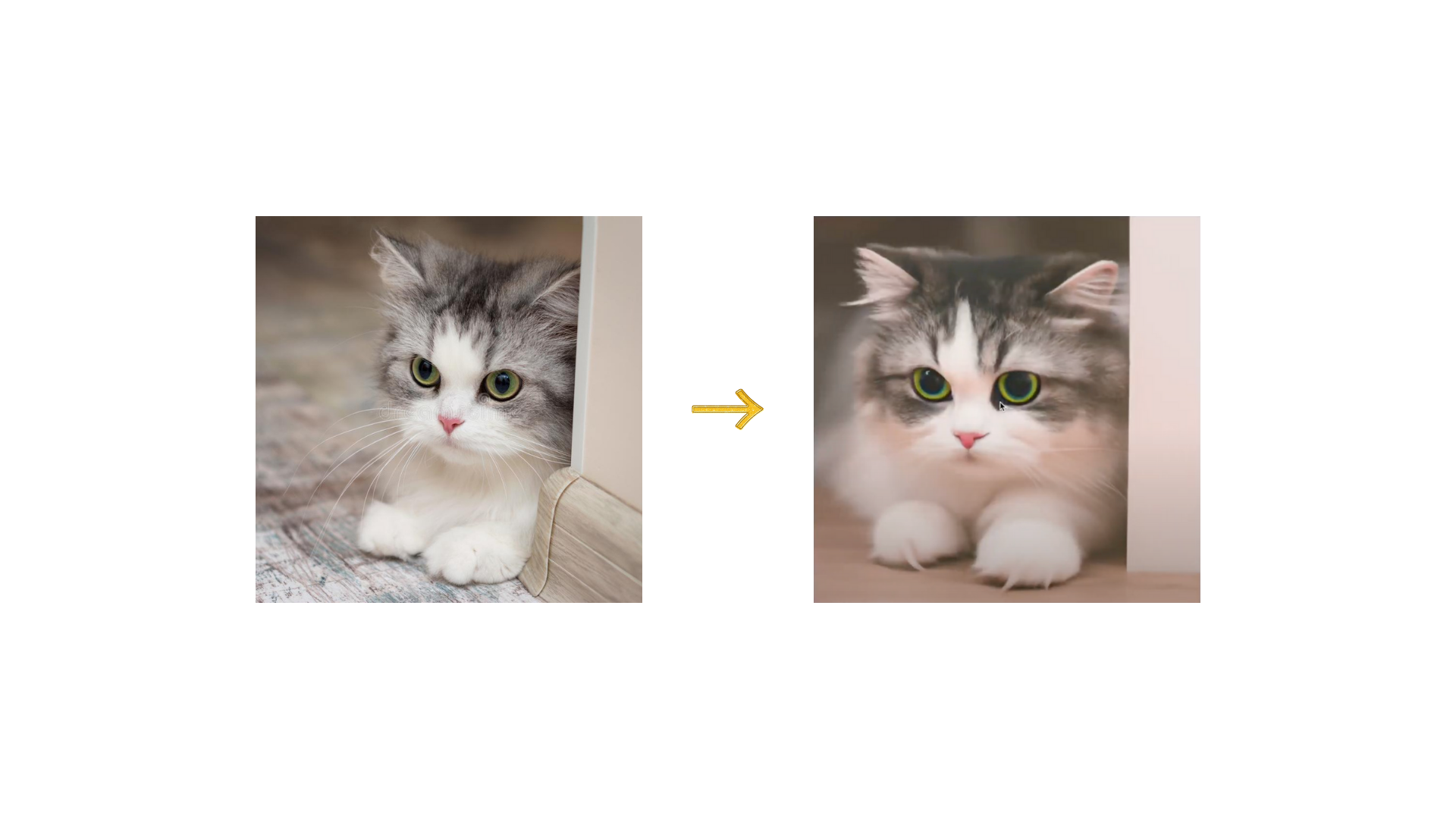
import openai import constants openai.api_key = constants.API_KEY response = openai.Image.create_variation( image=open("dalle-2 examples/cute_cat.png", "rb"), n=1, size="512x512", ) image_url = response["data"][0]["url"] # Print the response print(image_url)
Pretty interesting the variation it gives us; it also adds some detail to the nails! The code is shown above.
Conclusion
Overall it is pretty easy to get started with this package and it can provide some powerful methods to manipulate images! Let me know what you think and how it helped you in your project. Interested to see the use cases of this in the coming months.